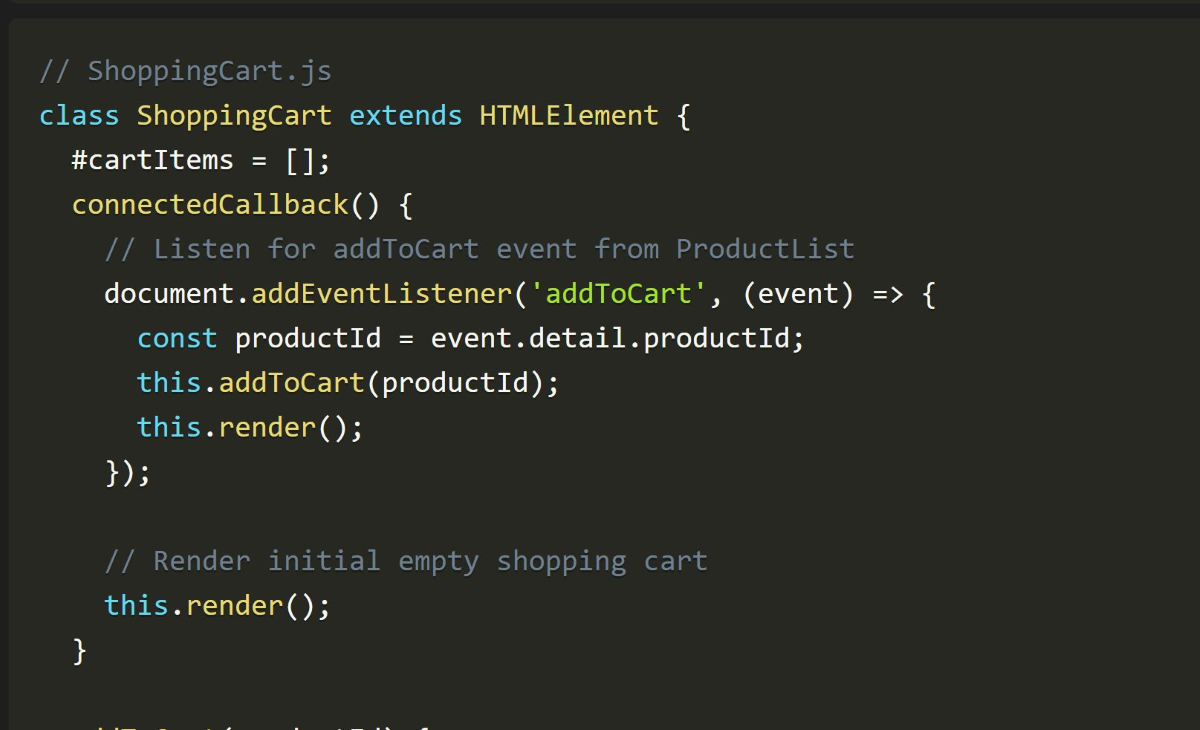
In the world of web development, HTML custom elements bring modularity and reusability to the forefront. They allow developers to encapsulate functionality within custom components. However, when it comes to orchestrating communication between these components, custom events play a pivotal role.
Defining Custom Elements
Before we delve into the intricacies of custom events, let's ensure that our custom elements are properly defined. Using the customElements.define() method, we register our elements with the browser.
| // ProductList.js
class ProductList extends HTMLElement {
connectedCallback() {
// Set up event listeners and render product list HTML
}
}
customElements.define('product-list', ProductList);
|
| // ShoppingCart.js
class ShoppingCart extends HTMLElement {
connectedCallback() {
// Set up event listeners and render shopping cart HTML
}
}
customElements.define('shopping-cart', ShoppingCart);
|
The Role of Custom Events
Custom events provide a means for these custom elements to communicate and react to changes. In our scenario, the ProductList component dispatches a custom event, addToCart, when a user clicks the "Add to Cart" button. The ShoppingCart component listens for this event and updates its state accordingly.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35 | // ProductList.js
class ProductList extends HTMLElement {
#products = [];
connectedCallback() {
this.render();
// Attach event listener for the "Add to Cart" button
this.querySelectorAll('.addToCart').forEach(button => {
button.addEventListener('click', event => this.handleAddToCart(event));
});
}
handleAddToCart(event) {
const productId = parseInt(event.target.dataset.id);
// Dispatch a custom event with the selected product
document.dispatchEvent(new CustomEvent('addToCart', { detail: { productId } }));
}
render() {
// Render product list HTML
this.innerHTML = `
<h2>Product List</h2>
<ul>
${this.#products.map(product => `
<li>
${product.name} - $${product.price}
<button class="addToCart" data-id="${product.id}">Add to Cart</button>
</li>`).join('')}
</ul>`;
}
}
customElements.define('product-list', ProductList);
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36 | // ShoppingCart.js
class ShoppingCart extends HTMLElement {
#cartItems = [];
connectedCallback() {
// Listen for addToCart event from ProductList
document.addEventListener('addToCart', (event) => {
const productId = event.detail.productId;
this.addToCart(productId);
this.render();
});
// Render initial empty shopping cart
this.render();
}
addToCart(productId) {
// Fetch product details based on productId (replace with your logic)
const product = { id: productId, name: `Product ${productId}`, price: 19.99 };
// Add the product to the shopping cart
this.#cartItems.push(product);
}
render() {
// Render shopping cart HTML
this.innerHTML = `
<h2>Shopping Cart</h2>
<ul>
${this.#cartItems.length === 0 ? '<li>Empty Cart</li>' :
this.#cartItems.map(item => `<li>${item.name} - $${item.price}</li>`).join('')
}
</ul>`;
}
}
customElements.define('shopping-cart', ShoppingCart);
|
Ensuring Event Handling in Shadow DOM
If one of the custom elements utilizes Shadow DOM, ensure that events inside the Shadow DOM are accessible from outside. Proper event handling within the Shadow DOM is crucial for seamless communication.
Conclusion
By embracing the synergy between HTML custom elements and custom events, developers can create modular and interconnected components. This approach promotes maintainability, scalability, and a cleaner architecture in web applications.
Feel free to experiment with these concepts and adapt them to your specific use cases. Custom elements and events empower developers to build flexible and robust web applications that stand the test of time. Happy coding! 🚀